Boring Bodies#
Description#
The BoringBodies
component represents a list of bodies falling through air, experiencing drag in all three axes as a primary result.
As aerodynamics of arbitrary bodies is difficult to simulate accurately, BoringBodies
simulates them by assuming that the velocities experienced any body can be resolved into the three principled axes (X, Y, Z), which then cause individual drag forces on the body in the same three axes (X, Y, Z).
The following figure illustrates this idea in 2D.
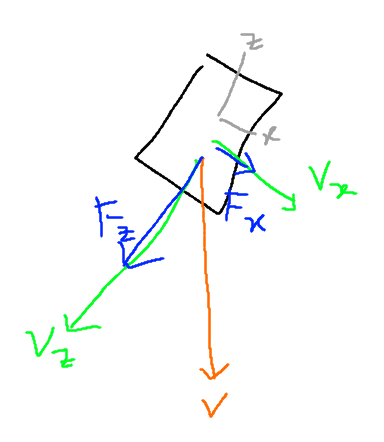
More concisely, the figure shows two reference frames — a ground frame \(XZ_G\) and a body frame \(XZ_B\). The velocity \(\mathbf{v}\) is resolved into body frame components \(v_x\), \(v_y\) (not shown in figure) and \(v_z\). The drag on the body itself is then computed using the familiar drag equation:
where:
\(i \in \{x, y, z\}\)
\(\rho\) is the density of air, uniformly assumed to be 1.225 kg/m^3
\(A\) is the frontal area in the direction of \(i\)
\(C_d\) is the drag coefficient in the direction of \(i\)
Class Description#
- class PyFlyt.core.abstractions.BoringBodies(p: BulletClient, physics_period: float, np_random: RandomState, uav_id: int, body_ids: ndarray | Sequence[int], drag_coefs: ndarray, normal_areas: ndarray)#
Vectorized implementation of a series of plain bodies affected by aerodynamics.
The BoringBodies component is used to represent a normal body moving through the air.
- Parameters:
p (bullet_client.BulletClient) – PyBullet physics client ID.
physics_period (float) – physics period of the simulation.
np_random (np.random.RandomState) – random number generator of the simulation.
uav_id (int) – ID of the drone.
body_ids (np.ndarray | Sequence[int]) – (n,) array of IDs for the links representing the bodies.
drag_coefs (np.ndarray) – (n, 3) array of drag coefficients for each body in the link-referenced XYZ directions.
normal_areas (np.ndarray) – (n, 3) array of frontal areas in the link-referenced XYZ directions.
- get_states()#
get_states does not exist for boring bodies, they’re boring.
- physics_update()#
Applies a force to the boring bodies depending on their local surface velocities.
- reset()#
Reset the boring bodies.
- state_update(rotation_matrix: ndarray)#
Updates the local surface velocity of the boring body.
- Parameters:
rotation_matrix (np.ndarray) – (3, 3) rotation_matrix of the main body