PyFlyt/Fixedwing-Waypoints-v1
#
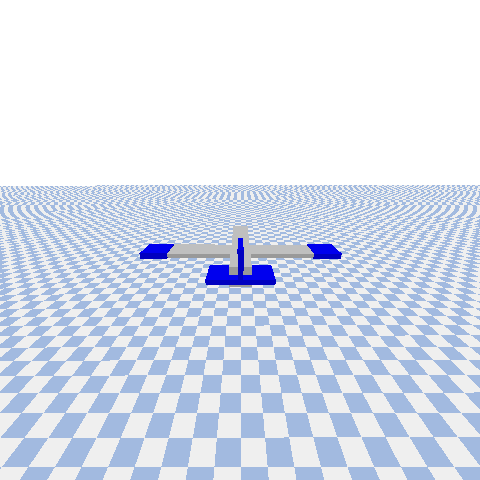
Task Description#
The goal of this environment is to fly a fixedwing aircraft towards a set of waypoints as fast as possible.
Usage#
import gymnasium
import PyFlyt.gym_envs
env = gymnasium.make("PyFlyt/Fixedwing-Waypoints-v1", render_mode="human")
term, trunc = False, False
obs, _ = env.reset()
while not (term or trunc):
obs, rew, term, trunc, _ = env.step(env.action_space.sample())
Flattening the Environment#
This environment uses the Dict
and Sequence
spaces from Gymnasium
, which are spaces with non-constant sizes.
This allows them to have complete observability without observation padding while making (human-)readability easier.
However, this results in them not being compatible with most popular reinforcement learning libraries like Stable Baselines 3 without custom wrappers.
If you would like to use this environment with those libraries, you can flatten the environment using the FlattenWaypointEnv
wrapper, where the argument context_length
specifies how many immediate targets are included in the observation.
import gymnasium
import PyFlyt.gym_envs
from PyFlyt.gym_envs import FlattenWaypointEnv
env = gymnasium.make("PyFlyt/Fixedwing-Waypoints-v1", render_mode="human")
env = FlattenWaypointEnv(env, context_length=2)
term, trunc = False, False
obs, _ = env.reset()
while not (term or trunc):
obs, rew, term, trunc, _ = env.step(env.action_space.sample())
Environment Options#
- class PyFlyt.gym_envs.fixedwing_envs.fixedwing_waypoints_env.FixedwingWaypointsEnv(sparse_reward: bool = False, num_targets: int = 4, goal_reach_distance: float = 2.0, flight_mode: int = 0, flight_dome_size: float = 100.0, max_duration_seconds: float = 120.0, angle_representation: Literal['euler', 'quaternion'] = 'quaternion', agent_hz: int = 30, render_mode: None | Literal['human', 'rgb_array'] = None, render_resolution: tuple[int, int] = (480, 480))#
Fixedwing Waypoints Environment.
Actions are roll, pitch, yaw, thrust commands. The target is a set of [x, y, z] targets in space
- Parameters:
sparse_reward (bool) – whether to use sparse rewards or not.
num_targets (int) – number of waypoints in the environment.
goal_reach_distance (float) – distance to the waypoints for it to be considered reached.
flight_mode (int) – The flight mode of the UAV.
flight_dome_size (float) – size of the allowable flying area.
max_duration_seconds (float) – maximum simulation time of the environment.
angle_representation (Literal["euler", "quaternion"]) – can be “euler” or “quaternion”.
agent_hz (int) – looprate of the agent to environment interaction.
render_mode (None | Literal["human", "rgb_array"]) – render_mode
render_resolution (tuple[int, int]) – render_resolution