PyFlyt/Rocket-Landing-v1
#
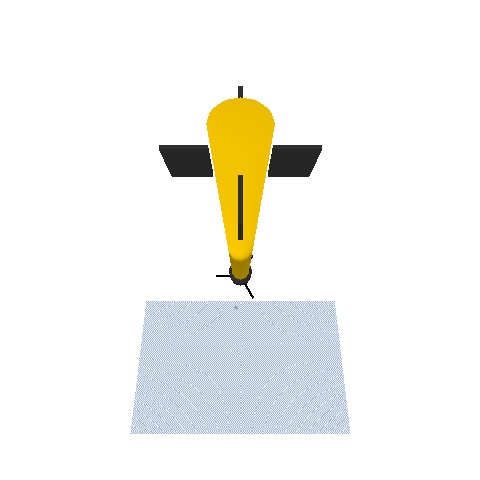
Task Description#
The goal of this environment is to land a rocket falling at terminal velocity on a landing pad, with only 1% of fuel remaining.
Usage#
import gymnasium
import PyFlyt.gym_envs
env = gymnasium.make("PyFlyt/Rocket-Landing-v1", render_mode="human")
term, trunc = False, False
obs, _ = env.reset()
while not (term or trunc):
obs, rew, term, trunc, _ = env.step(env.action_space.sample())
Environment Options#
- class PyFlyt.gym_envs.rocket_envs.rocket_landing_env.RocketLandingEnv(sparse_reward: bool = False, ceiling: float = 500.0, max_displacement: float = 200.0, max_duration_seconds: float = 30.0, angle_representation: Literal['euler', 'quaternion'] = 'quaternion', agent_hz: int = 40, render_mode: None | Literal['human', 'rgb_array'] = None, render_resolution: tuple[int, int] = (480, 480))#
Rocket Landing Environment.
Actions are finlet_x, finlet_y, finlet_roll, booster ignition, throttle, booster gimbal x, booster gimbal y The goal is to land the rocket on the landing pad.
- Parameters:
sparse_reward (bool) – whether to use sparse rewards or not.
ceiling (float) – the absolute ceiling of the flying area.
max_displacement (float) – the maximum horizontal distance the rocket can go.
max_duration_seconds (float) – maximum simulation time of the environment.
angle_representation (Literal["euler", "quaternion"]) – can be “euler” or “quaternion”.
agent_hz (int) – looprate of the agent to environment interaction.
render_mode (None | Literal["human", "rgb_array"]) – render_mode
render_resolution (tuple[int, int]) – render_resolution.